Background
The Sum of all fears coding challenge is intended to assess a candidate’s ability to work with Arrays. This challenge or a slight variation has been used by technology companies such as Amazon and Google and was also featured as a Daily Coding Problem in dev.to.
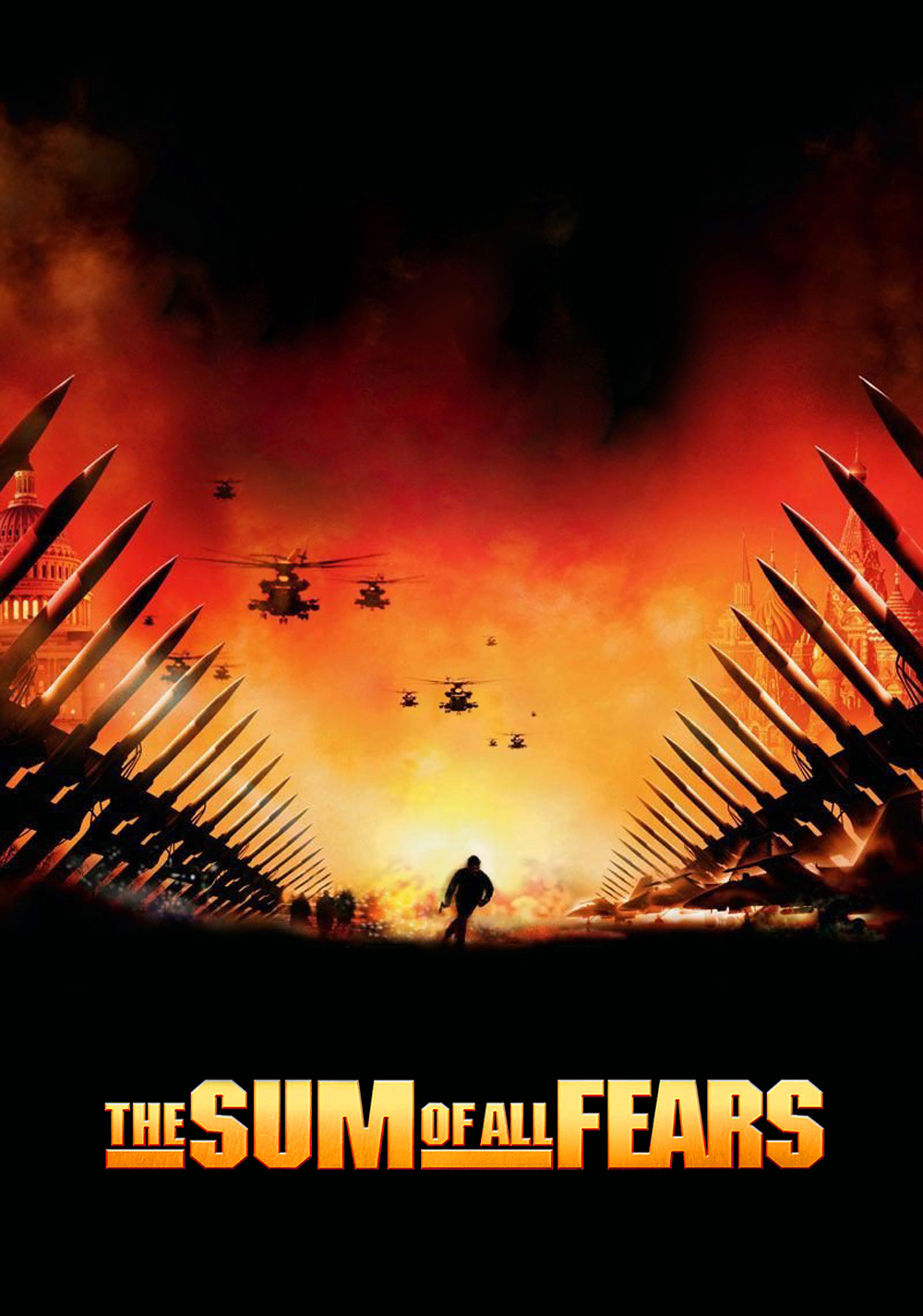
Description
Given a list of numbers and a number K, return whether any two numbers from the list add up to K.
Example: given [10, 15, 3, 7] and K of 17, return true since 10 + 7 is 17.
The basic challenge is to write a program that uses a hard-coded array of [10, 15, 3, 7] and allows the user to enter a value for K before running the algorithm and returning true or false.
Solve
Note: Each button press will run code for each click, for each click a randomly generated array of numbers will run. Default result will be false until a solution is found.
Results:
Code
The following is my JavaScript code used for this exercise. Read over if your interested.
Technologies
Technologies Used: HTML, CSS, JavaScript, jQuery and Bootstrap. Made in VS Studio 2019 with code on GitHub.
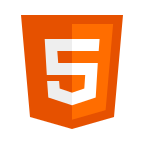
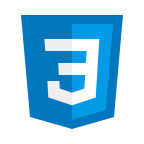
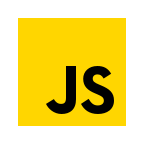
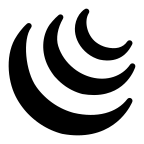
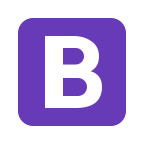

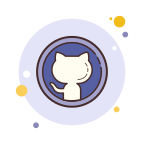